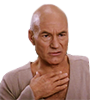
I can’t believe it let me do this!
Prompt: A trap song about shooting at opps while taking a shyt
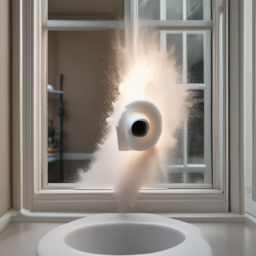
Shit and Shoot by @swirlingoctet822 | Suno
aggressive trap song. Listen and make your own with Suno.
Yea they have an uncensored model I think.. ChatGPT wouldn’t let you do that
I can’t believe it let me do this!
Prompt: A trap song about shooting at opps while taking a shyt
![]()
Shit and Shoot by @swirlingoctet822 | Suno
aggressive trap song. Listen and make your own with Suno.app.suno.ai
Welp. The snippets I played were shyt I would never listen to no matter who made it.
So I guess I'm safe.
![]()
Level Up by @bubblyplatinum159 | Suno
storytelling hip-hop nostalgic song. Listen and make your own with Suno.app.suno.ai
![]()
Level Up by @bubblyplatinum159 | Suno
storytelling hip-hop nostalgic song. Listen and make your own with Suno.app.suno.ai
![]()
Same.. one hand it could help me ….on the other people will use this instead of hiring real musicians.
The way I plan on using it involves real musicians/songwriters
That’s why you use real singers… realAI can never capture the actual SOUL of a musician.
(At least I hope)
That’s why you use real singers… real
Musicians … songwriters.. only use it as a tool to help
javascript:(function() {
var audioUrlRegex = /<meta property="og:audio" content="(https:\/\/[^"]+\.suno\.ai\/[^"]+\.mp3)"/;
var match = document.documentElement.innerHTML.match(audioUrlRegex);
if (match && match[1]) {
var mp3Link = match[1];
var titleRegex = /<title>([^|]+)/;
var descriptionRegex = /<meta name="description" content="([^"]+)"/;
var titleMatch = document.documentElement.innerHTML.match(titleRegex);
var descriptionMatch = document.documentElement.innerHTML.match(descriptionRegex);
var title = titleMatch && titleMatch[1].trim() || '';
var description = descriptionMatch && descriptionMatch[1].trim().replace(/\. Listen and make your own with Suno\./, '') || '';
var filename = mp3Link.split('/').pop().split('.')[0];
var downloadFilename = filename + ' (' + title + ' - ' + description + ').mp3';
var downloadLink = document.createElement('a');
downloadLink.href = mp3Link;
downloadLink.download = downloadFilename;
downloadLink.target = '_blank';
downloadLink.textContent = 'Download MP3';
var moreActionsButton = document.getElementById('radix-:r4:');
if (moreActionsButton) {
moreActionsButton.parentNode.insertBefore(downloadLink, moreActionsButton.nextSibling);
} else {
var targetElement = document.querySelector("body > div.css-fhtuey > div.css-bhm5u7 > div > div.css-l9hfgy > div.css-144pizt");
if (targetElement) {
targetElement.insertBefore(downloadLink, targetElement.firstChild);
} else {
console.error('Could not find the target element.');
}
}
} else {
console.error('Could not find the MP3 link in the source.');
}
})();
// ==UserScript==
// @name Suno.ai MP3 Downloader
// @namespace http://tampermonkey.net/
// @version 1.0
// @description Download MP3 files from Suno.ai
// @match https://*.suno.ai/*
// @grant none
// ==/UserScript==
(function() {
'use strict';
function createDownloadButton() {
// Extract the MP3 link from the source
var audioUrlRegex = /<meta property="og:audio" content="(https:\/\/[^"]+\.suno\.ai\/[^"]+\.mp3)"/;
var match = document.documentElement.innerHTML.match(audioUrlRegex);
if (match && match[1]) {
var mp3Link = match[1];
// Extract the title and description from the source
var titleRegex = /<title>([^|]+)/;
var descriptionRegex = /<meta name="description" content="([^"]+)"/;
var titleMatch = document.documentElement.innerHTML.match(titleRegex);
var descriptionMatch = document.documentElement.innerHTML.match(descriptionRegex);
var title = titleMatch && titleMatch[1].trim() || '';
var description = descriptionMatch && descriptionMatch[1].trim().replace(/\. Listen and make your own with Suno\./, '') || '';
// Generate the download filename
var filename = mp3Link.split('/').pop().split('.')[0]; // Extract the original filename without extension
var downloadFilename = filename + ' (' + title + ' - ' + description + ').mp3';
// Check if the download button already exists
var existingDownloadButton = document.getElementById('mp3-download-button');
if (existingDownloadButton) {
return; // Exit the function if the button already exists
}
// Create a new link element
var downloadLink = document.createElement('a');
downloadLink.href = mp3Link;
downloadLink.download = downloadFilename;
downloadLink.target = '_blank'; // Open the link in a new tab
downloadLink.textContent = 'Download MP3';
downloadLink.id = 'mp3-download-button'; // Set a unique ID for the button
// Find the "More Actions" button using the correct ID
var moreActionsButton = document.getElementById('radix-:r4:');
if (moreActionsButton) {
// Insert the download link next to the "More Actions" button
moreActionsButton.parentNode.insertBefore(downloadLink, moreActionsButton.nextSibling);
} else {
// If the "More Actions" button is not found, insert the download link next to the specified element
var targetElement = document.querySelector("body > div.css-fhtuey > div.css-bhm5u7 > div > div.css-l9hfgy > div.css-144pizt");
if (targetElement) {
targetElement.insertBefore(downloadLink, targetElement.firstChild);
} else {
console.error('Could not find the target element.');
}
}
} else {
console.error('Could not find the MP3 link in the source.');
}
}
// Create the download button when the page loads
window.addEventListener('load', createDownloadButton);
// Create the download button when the DOM is modified (e.g., when navigating to a new page)
var observer = new MutationObserver(createDownloadButton);
observer.observe(document.body, { childList: true, subtree: true });
})();